Since this image was relatively large, and therefore would make octave run slowly, I reduced the image size to 300x287 and saved it as a png file.
I then used photoshop to sharpen the image using the filter/sharpen.../unsharp mask... tool; using Amount=291, Radius=2.3, and Threshold=0. If I made the radius any bigger, then some of the bricks would start to get very dark and the 'vertical' lines would get thicker and darker. If I increase the Threshold even slightly, then the 'main' bricks on building become blurry. This is not what I am looking for as I want the brick lines/detail to pop out more.
Here is the original picture followed by the 'unsharp masked' imaged.
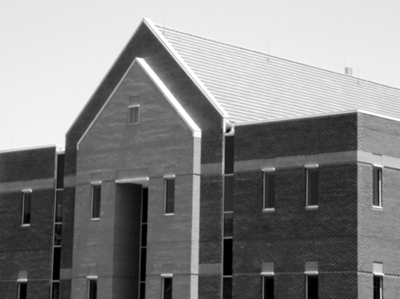
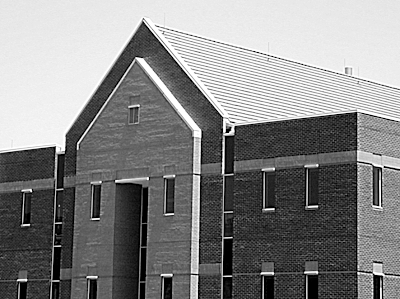
As you can observe, the 'fixed' building looks much clearer, with more brick lines visible and crisp.
Using octave I tried to mimic this clarity by using sharpening codes for Laplacian in 2 and 4 directions, and the method of unsharp masking. I will then compare and contrast these methods.
I will start with the Laplacian sharpening method. The octave code I used was:
# Laplacian with 2 directions...
a=imread("building_blur.png");
b=double(a)/255;
w4=fspecial("laplacian",0);
g4=b-imfilter(b,w4,'replicate');
imshow(g4);
# Note that you can use values (Real) between 0 and 1 in this code for the
w4=fspecial("laplacian",0) line.
Here is how the building with Laplacian at 0, 0.5, and 1, respectively looks like.
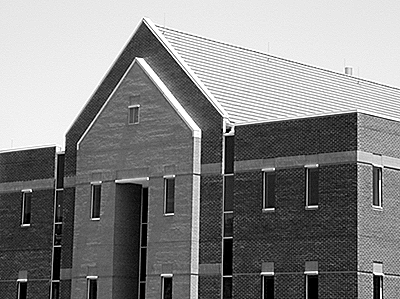
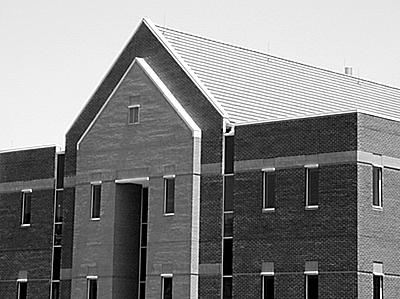
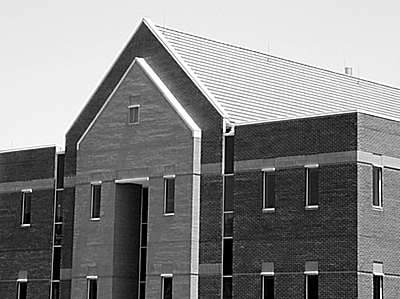
The pictures all look similar with respect to how they enhance the 'brick lines', however, if you look at the 'awning' or the 'triangular roof' that is on the actual brickwork, you will notice that above it a slight 'white smudge' can be noticed above the roof, on the brickwork as the values increase from 0 to 1. Also, it appears that this Laplacian value, as it increases, provides a sort of whitening effect, much in the same way the 'amount' value does in photoshop when using the unsharp masking tool. In any event, the blurred building looks much sharper after applying the Laplacian 2-direction octave code.
Here is how the Laplacian 4-direction code affects the building_blur.tif file:
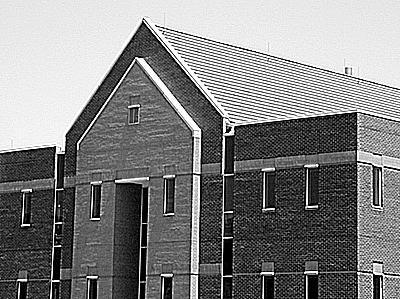
As you can see, the building which had the laplacian applied to it for: horizontal, vertical, and diagonal; shows even 'finer' detail. Namely, you can see the actual brick lines along the horizontal and vertical directions. In the previous building pictures which only used the Laplacian in the horizontal and vertical directions, the 'vertical brick lines' were not as noticeable. Also, compare the vertical window lines of the 4-dir laplacian picture and the other three 2-dir laplacian pictures. You will notice that the 4-dir windows look much crisper and stand out more. The 4-dir laplacian building also looks "lighter/whiter" than the other pictures. I think this can be attributed to the increased detail evident in the 4-dir laplacian picture.
Additionally, the lines on the 'roof' stand out much more and look dramatic in comparison to the other 'fixed' images.
The octave code I use for the 4-dir Laplacian was:
# Laplacian with 4 directions...
a=imread("building_blur.png");
b=double(a)/255;
w8 = [1, 1, 1; 1, -8, 1; 1, 1, 1];
g8 = b - imfilter(b, w8, 'replicate');
imwrite("building_4dir.png",g8);
So now, we have 3 'fixed images' for the 'blurred building'. Let's see how the blurred image would look like if I use the 'masking technique'. That is, the process whereby I first blur the image, create a mask by subtracting the blurred image from the original image, and then create a new image by adding the product of some value 'k' multiplied by the masking process, to the original image.
Here is the octave code I used:
a=imread("building_blur.png");
b=double(a)/255;
# set up gaussian blurring...
G=fspecial('gaussian',[3,3],0.5);
# blur the image now...
c = imfilter(b,G);
# make a 'mask'...
gmask=b-c;
# make a new 'g' with a large k value...
k=15;
g=b+(k*gmask);
Here is the resulting image...
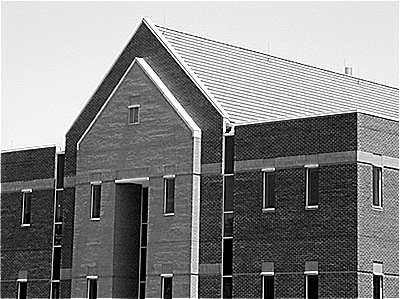
Now, while the bricks on the building actually look better in terms of providing finer detail...you can see that the sky has a bit of noise visible there. We need to fix this. Let's try increasing k=20.
Here is the resulting image...
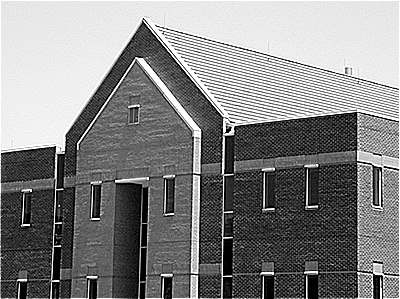
I notice that the building has even finer detail evident in the brickwork and that the sky doesn't look as 'noisy'...but let's see if we can do better by increasing the value of sigma in the gaussian blur tool. I choose to do this because we learned that if you increase sigma in a gaussian blur, the image will be blurred more. For consistency I will leave k=20.
Here is the image with sigma=1 and k=20:
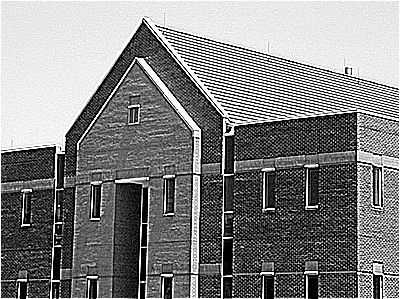
Well, as you can see, the building has increased detail, however the sky similarly has increased 'noise'.
To fix this, let's try applying a threshold of th=5 to our existing code.
a=imread("building_blur.png");
b=double(a)/255;
# set up gaussian blurring...
G=fspecial('gaussian',[3,3],1);
# blur the image now...
c = imfilter(b,G);
# make a 'mask'...
gmask=b-c;
# make a new 'g' with a large k value...
k=20;
# threshold...
th=5;
ind=find(gmask<=th);
gmask(ind)=0;
g=b+(k*gmask);
imwrite("build_th5_k20.png", g);
The image looks like this:
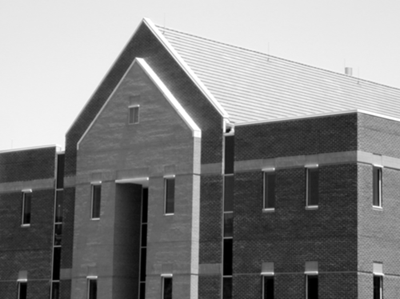
Well, this image looks pretty good in the sense that the sky now looks uniform and the 'noise' is not as prominent in the sky. However, the brickwork detail i.e. the lines are not as obvious anymore and the fine detail is missing, owing to the 'smudging' factor of the th=5.
Let's see if we can have the sky 'less noisy' but the brick work more obvious.
Let th=1 and k=20.
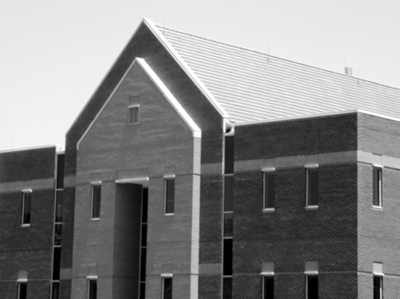
I do not detect that much of a difference...Let us try for th=1 and k=30.
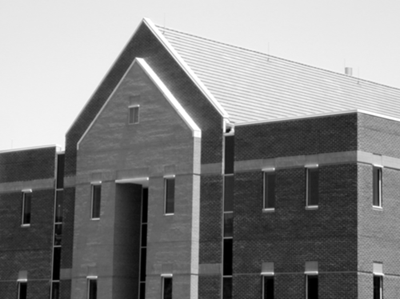
Again, the image does not look any better. The window at the top of the building looks a little blurry to me.
In my opinion, the images that were created with k=20 and th=1,5 are the best images if you are using unmask sharpening, with a threshold factor greater than 0.
They each take care of the noise problem in the sky, they provided finer detail in the brickwork, and they highlight the edges; thereby giving a sharper image. However, if you are looking for finer detail in the building and care about the 'noise' in the sky to a lesser extent, then I would use the unmask sharpening technique where th=0. Even so, if I had to choose between either method, I would probably use the Laplacian sharpening method in 2-directions (using value= 0.5) instead of the 'unmask sharpening' method, with or without threshold.